@property
: CSS variables on steroids
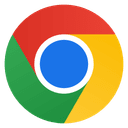
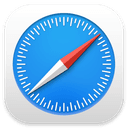
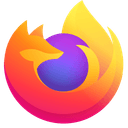
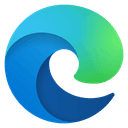
CSS variables are great, but they have some limitations. Mainly, they are all treated as strings. @property
gives your CSS variables semantic meaning and default values.
There are two ways to define a custom property. You can do it via JavaScript:
CSS.registerProperty({
name: "--boxSize",
syntax: "<length>",
initialValue: "100px",
inherits: false,
});
Or you can do the same in CSS directly:
@property --boxSize {
syntax: "<length>";
initial-value: "100px";
inherits: false;
}
There are many options you can choose for syntax
. Some are: length
, number
, percentage
, color
, image
, and url
. Check the links below for a complete list.
The effect of defining a custom property in this way is that now your browser knows what type of value it should expect. If you try to assign a number to a color property, it will ignore it:
@property --primaryColor {
syntax: "<color>";
initial-value: "rebeccapurple";
inherits: false;
}
.wrongBox {
--primaryColor: 100px; /* ignored */
background: var(--primaryColor); /* rebeccapurple */
}
.rightBox {
--primaryColor: blue;
background: var(--primaryColor); /* blue */
}
Another handy use case is adding a transition to a linear gradient. Currently, this is not possible:
.box {
--percentage: 20%;
background: linear-gradient(red var(--percentage), transparent);
transition: background 0.5s;
}
.box:hover {
--percentage: 100%;
}
The code above won’t work because the browser doesn’t know that --percentage
is a number and will try to create a transition between two strings. The solution is to define --percentage
using @property
:
@property --percentage {
syntax: "<percentage>";
initial-value: 20%;
inherits: false;
}
.box {
background: linear-gradient(red var(--percentage), transparent);
transition: --percentage 0.5s;
}
.box:hover {
--percentage: 100%;
}